ShaderHandler
ShaderInterface
Availability LightWave 6.0 | Component Layout, Modeler | Header lwshader.h
Shaders set the color and other appearance attributes of each visible spot on a surface.
A surface, sometimes called a material in other programs, is a collection of attributes that define the appearance of a polygon. The same surface can be applied to multiple objects, and different surfaces can be applied to different polygons on the same object. Shaders are always associated with a surface and affect its appearance during rendering by setting or modifying its attributes. More than one shader can be associated with a surface, and the effects of one shader might in turn be modified by the next shader in line. A shader can also fire rays into the scene that cause other shaders to be evaluated.
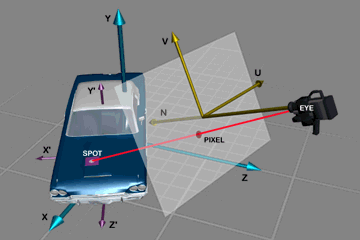
For each pixel in the rendered image, the renderer finds the spot in the scene that the camera sees at that pixel. If the spot is on an object, its appearance depends on the suface assigned to the polygon it lies in. The renderer uses the surface settings to calculate a color for the pixel, and if a shader is attached to the surface, its evaluation function is called to either modify the surface settings or perform its own color calculation.
The shader evaluation function is given the surface attributes, the geometry of the spot, the ID of the object and the polygon, the source of the viewing ray, and other contextual information, and it has access to raytracing functions that can tell it even more about the scene.
Handler Activation Function
XCALL_( int ) MyShader( long version, GlobalFunc *global, LWShaderHandler *local, void *serverData );
The local
argument to a shader's activation function is an LWShaderHandler.
typedef struct st_LWShaderHandler { LWInstanceFuncs *inst; LWItemFuncs *item; LWRenderFuncs *rend; void (*evaluate) (LWInstance, LWShaderAccess *); unsigned int (*flags) (LWInstance); } LWShaderHandler;
The first three members of this structure are the standard handler functions. In addition to these, a shader provides an evaluation function and a flags function.
The context
argument to the create
function is the LWSurfaceID for
the surface. LWSurfaceID is defined in the lwsurf.h
header file and is used by
the surface functions global.
A shader can be activated by Modeler as well as Layout. When activated by Modeler, the
LWItemFuncs pointer in the local data is NULL. Be sure to test for this before filling in
the useItems
and changeID
fields. Note too that if your shader relies on
Layout-only globals, those won't be available when Modeler calls your callbacks.
evaluate( instance, access )
- This is where the shader does its work. At each time step in the animation, the evaluation function is called for each pixel affected by the shader's surface. The access argument, described below, contains information about the spot to be colored.
flagbits = flags( instance )
- Returns an int that tells the renderer which surface attributes the shader will modify
and whether it will call the raytracing functions. The flags are bits combined using
bitwise-or. They correspond to members of the shader access structure described below. For
efficiency reasons, the renderer may ignore changes to any surface attributes that weren't
indicated by the bit flags returned from this function, and it won't provide access to the
raytracing functions unless the
LWSHF_RAYTRACE
bit is set. The flags areLWSHF_NORMAL
LWSHF_COLOR
LWSHF_LUMINOUS
LWSHF_DIFFUSE
LWSHF_SPECULAR
LWSHF_MIRROR
LWSHF_TRANSP
LWSHF_ETA
LWSHF_ROUGH
LWSHF_TRANSLUCENT
LWSHF_RAYTRACE
Interface Activation Function
XCALL_( int ) MyInterface( long version, GlobalFunc *global, LWInterface *local, void *serverData );
This is the standard interface activation for handlers. Inline xpanels will appear in the Shaders tab of the Surface Editor.
Shader Access
The evaluation function is called for every visible spot on a surface and is passed a shader access structure describing the spot to be shaded. The access structure contains some values which are read-only and some which are meant to be modified. The read-only values describe the geometry of the pixel being shaded. The read-write values describe the current attribute settings of the spot and should be modified in place to affect the final look of the spot. Since shaders may be layered, these properties may be altered many more times before final rendering. The access structure also contains raytracing functions that can be called only while rendering.
typedef struct st_LWShaderAccess { int sx, sy; double oPos[3], wPos[3]; double gNorm[3]; double spotSize; double raySource[3]; double rayLength; double cosine; double oXfrm[9], wXfrm[9]; LWItemID objID; int polNum; double wNorm[3]; double color[3]; double luminous; double diffuse; double specular; double mirror; double transparency; double eta; double roughness; LWIlluminateFunc *illuminate; LWRayTraceFunc *rayTrace; LWRayCastFunc *rayCast; LWRayShadeFunc *rayShade; int flags; int bounces; LWItemID sourceID; double wNorm0[3]; double bumpHeight; double translucency; double colorHL; double colorFL; double addTransparency; double difSharpness; LWPntID verts[4]; float weights[4]; float vertsWPos[4][3]; LWPolID polygon; double replacement_percentage; double replacement_color[3]; double reflectionBlur; double refractionBlur; } LWShaderAccess;
Read-Only Parameters
These fields provide read-only information about the local geometry of the spot and the context of the evaluation.
sx, sy
- The pixel coordinates at which the spot is visible in the rendered image. This is labeled PIXEL in the figure, but note that it won't necessarily be the spot's projection onto the viewplane. When the viewing ray originates on a reflective surface, for example, the pixel coordinates are usually for the source of the ray (the spot's reflection). The pixel coordinate origin (0, 0) is in the upper left corner of the image.
oPos
- Spot position in object (Modeler) coordinates (the (X', Y', Z') system in the figure).
wPos
- Spot position in world coordinates (X, Y, Z). This is the position after transformation and the effects of bones, displacement and morphing.
gNorm
- Geometric normal in world coordinates. This is the raw polygonal normal at the spot, unperturbed by smoothing or bump mapping.
wNorm0
- The interpolated normal in world coordinates. This is the same as
gNorm
, but after smoothing. spotSize
- Approximate spot diameter, useful for texture antialiasing. The diameter is only approximate because spots in general aren't circular. On a surface viewed on edge, they're long and thin.
raySource
- Origin of the incoming viewing ray in world coordinates. Labeled EYE in the figure, this is often the camera, but it can also, for example, be a point on a reflective surface.
rayLength
- The distance the viewing ray traveled in free space to reach this spot (ordinarily the
distance between
raySource
andwPos
). cosine
- The cosine of the angle between the raw surface normal and a ray pointing from the spot
back toward the
raySource
. This is the same as the dot product ofgNorm
and the unit vector(raySource - wPos)/rayLength
. Low values correspond to high angles and therefore glancing views. This is also a measure of how approximate the spot size is. oXfrm
- Object to world transformation matrix. The nine values in this array form a 3 x 3 matrix
that describes the rotation and scaling of the object. This is useful primarily for
transforming direction vectors (bump gradients, for example) from object to world space.
LWDVector ovec, wvec; wvec[ 0 ] = ovec[ 0 ] * oXfrm[ 0 ] + ovec[ 1 ] * oXfrm[ 1 ] + ovec[ 2 ] * oXfrm[ 2 ]; wvec[ 1 ] = ovec[ 0 ] * oXfrm[ 3 ] + ovec[ 1 ] * oXfrm[ 4 ] + ovec[ 2 ] * oXfrm[ 5 ]; wvec[ 2 ] = ovec[ 0 ] * oXfrm[ 6 ] + ovec[ 1 ] * oXfrm[ 7 ] + ovec[ 2 ] * oXfrm[ 8 ];
wXfrm
- World to object transformation matrix (the inverse of
oXfrm
). objID
- The object being shaded. It's possible for a single shader instance to be shared between multiple objects, so this may be different for each call to the shader's evaluation function. For sample sphere rendering the ID will refer to an object not in the current scene.
polNum
- An index identifying the polygon that contains the spot. It may represent other
sub-object information in non-mesh objects. See also the
polygon
field. flags
- Bit fields describing the nature of the call. The
LWSAF_SHADOW
bit tells you when the evaluation function is being called during shadow computations, which you might want to treat differently from "regular" shader evaluation. bounces
- The number of times the viewing ray has branched, or bounced, before reaching this spot. This value can be used to limit recursion, particularly the shader's own calls to the raytracing functions.
sourceID
- The item ID of the source of the viewing ray.
verts
- The four vertices surrounding the spot, useful for interpolating vertex-based surface data.
weights
- The weights assigned to the four neighboring vertices.
vertsWPos
- The world coordinate position of the neighboring vertices.
polygon
- The polygon ID of the polygon containing the spot.
Modifiable Parameters
These parameters are used by the renderer to compute the perceived color at the spot and may be modified by the shader. Almost all of them correspond directly to surface parameters in the user interface, although the values may be represented by different ranges. Unless stated otherwise, the values of these fields nominally range from 0.0 to 1.0, and values outside that range are also valid.
The shader's flags function must have returned the correct flags for the fields the
shader will modify, or changes to these fields may be ignored. Prior to LightWave 7.0, to
set the perceived color directly a shader would set all of the parameters to zero except
for luminous
, which would be 1.0, and color
, which would be the output
color of the spot. Newer shaders can instead use the replacement_color
and replacement_percentage
fields.
wNorm
- Surface normal in world coordinates. If you modify this vector, you must renormalize it (make its length equal to 1.0).
bumpHeight
- The relative height of peaks in the bump map. Increasing this value makes the bump mapping appear more pronounced. Currently this is used solely as a texture input. If no texture is applied to the bump channel of the surface, it will be 0, and values you write will be ignored.
color
- The RGB components of the base color of the spot.
luminous
- Luminosity level. Higher values add more of the base color to the final color of the spot. This component is unaffected by lighting and shading.
diffuse
- Diffuse reflection level.
specular
- Specular reflection level.
mirror
- Mirror reflection level.
transparency
- Transparency level.
eta
- Index of refraction. In the real world this ranges between 1.0 and about 3.5, depending on the material, but values outside that range are also valid here.
roughness
- Surface roughness, the inverse of the exponent in the Phong specular highlight formula. The corresponding user parameter is called Glossiness. The roughness is approximately 2(10g+2).
translucency
- Translucency level. This determines how much the brightness of a surface is affected by the brightness of the environment behind it.
colorHL
- Amount of highlight coloring. Higher values mix more of the base color of the spot into the color of specular highlights, a simple way to simulate the behavior of metallic (nondielectric) surfaces.
colorFL
- Color filtering amount. This controls how strongly the light passing through a transparent surface is colored by that surface, which affects the color of other surfaces illuminated by this light.
addTransparency
- Additive transparency. An additively transparent surface adds its own color to the colors of surfaces seen through it. This usually has the effect of lightening the color of the underlying surfaces.
difSharpness
- Diffuse sharpness level. This controls how the shading varies with the angle of the light. Higher values make the brightness of illuminated areas more uniform and increase the sharpness of the transition between lit and dark areas (the terminator of a planet, for example).
replacement_percentage
replacement_color- Use these together to set a color for the surface that will be unaffected by subsequent shading and lighting calculations. This is typically used by plug-ins that partially replace LightWave's lighting model.
reflectionBlur
refractionBlur- The amount of blurring applied to reflections and refractions.
Rendering Functions
lit = illuminate( lightID, position, direction, color )
len = rayTrace( position, direction, color )
len = rayCast( position, direction )
len = rayShade( position, direction, shaderAccess )
History
LightWave 7.0 added the replacement_percentage
, replacement color
, reflectionBlur
and refractionBlur
fields to LWShaderAccess, but LWSHADER_VERSION
was not
incremented. If your shader activation accepts version 4, use the Product Info global to determine whether these fields
are available before attempting to read or write them.
Example
The blotch sample is a simple shader that renders a circular blotch of a user-specified position, size and color.